Examples of PHP Programs to Create Login and Logout Pages using Sessions
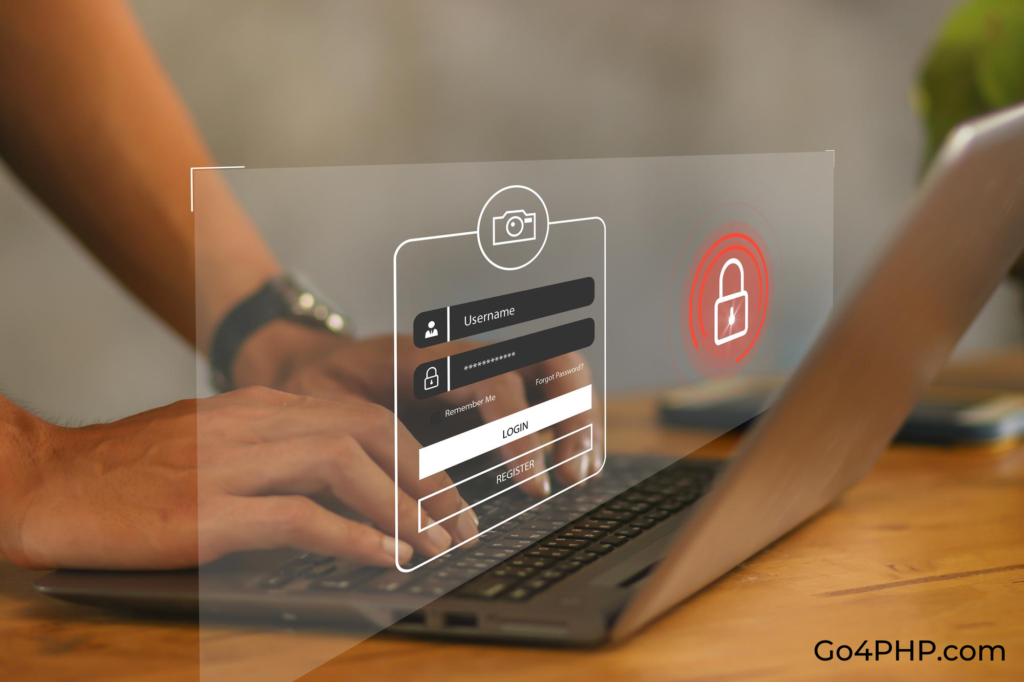
PHP is one of the most widely used programming languages since its inception back in 1995. Once you master it, you can fuel up your career as one of the best web developers. However, for newbies, understanding the concepts of PHP programs can be a little tricky. Particularly, sessions and cookies are two of the most tricky concepts to master as a PHP beginner.
While both concepts are related to data management, the significant difference between them is that sessions store user data in the server, while cookies store it in the browser. Session data can only be accessed during the current session.
In PHP 8, a user session starts with a successful login and ends with a logout. These two sessions are essential for any website. So, in this tutorial, we will be going to share with you examples of PHP programs to create login and logout using sessions. Let’s first understand the importance of sessions in website development.
- Sessions allow accessibility to user data across the entire website.
- Session data is saved on a temporary file in a server temporary directory – php.ini file.
- It automatically destroys the data when the user closes the browser.
PHP Programs Steps or Creating Login and Logout
- When a user logs in successfully, create a session variable to store their information.
- On the home page, check if the session variable is set or not. If it is not set, redirect the user to the login page.
- When a user logs out, destroy the session variable to clear their information.
Examples of PHP Programs for Creating Login and Logout Pages Using Session
Login Page
login.php
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> <title>Login Form</title> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css"> <script src="https://code.jquery.com/jquery-3.5.1.min.js"></script> <script src="https://cdn.jsdelivr.net/npm/popper.js@1.16.0/dist/umd/popper.min.js"></script> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/js/bootstrap.min.js"></script> <style> .login-form { width: 340px; margin: 50px auto; font-size: 15px; } .login-form form { margin-bottom: 15px; background: #f7f7f7; box-shadow: 0px 2px 2px rgba(0, 0, 0, 0.3); padding: 30px; } .login-form h2 { margin: 0 0 15px; } .form-control, .btn { min-height: 38px; border-radius: 2px; } .btn { font-size: 15px; font-weight: bold; } </style> </head> <body> <div class="login-form"> <form name="frmUser" method="post" action="loginProcess.php" align="center"> <h2 class="text-center">Log in</h2> <div class="message text-danger"><?php if($message!="") { echo $message; } ?></div> <div class="form-group"> <input type="text" class="form-control" name="username" placeholder="Username" required="required" autocomplete="off"> </div> <div class="form-group"> <input type="password" autocomplete="off" class="form-control" placeholder="Password" name="password" required="required"> </div> <div class="form-group"> <button type="submit" class="btn btn-primary btn-block" value="Submit">Log in</button> </div> </form> </div> </body> </html>
Login Process
loginProcess.php <?php session_start(); $message=""; if(count($_POST)>0) { $con = mysqli_connect('localhost','root','root','login') or die('Unable To connect'); $result = mysqli_query($con,"SELECT * FROM user WHERE username='" . $_POST["username"] . "' and password = '". $_POST["password"]."'"); $row = mysqli_fetch_array($result); if(is_array($row)) { $_SESSION["id"] = $row['id']; $_SESSION["name"] = $row['name']; } else { $message = "Invalid Username or Password!"; } } if(isset($_SESSION["id"])) { header("Location:index.php"); } ?> After the successfully login to the session then redirect to the index.php file.
Index Page
index.php <?php session_start(); ?> <!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> <title>Login Success</title> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css"> <script src="https://code.jquery.com/jquery-3.5.1.min.js"></script> <script src="https://cdn.jsdelivr.net/npm/popper.js@1.16.0/dist/umd/popper.min.js"></script> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/js/bootstrap.min.js"></script> <style> .login-form { width: 340px; margin: 50px auto; font-size: 15px; } </style> </head> <body> <div class="login-form"> <?php if($_SESSION["name"]) { ?> Welcome <?php echo $_SESSION["name"]; ?>. Click here to <a href="logout.php" tite="Logout">Logout. <?php }else echo "<h1>Please login first .</h1>"; ?> </div> </body> </html>
Logout Page
logout.php <?php session_start(); unset($_SESSION["id"]); unset($_SESSION["name"]); header("Location:login.php"); ?>
Conclusion
In this tutorial, we have provided some sample codes for the PHP programs to create login and logout pages using the sessions. Hope this guide helped you understand the topic. Login and logout sessions are essential elements of the PHP programs. Therefore, having a good understanding of these PHP elements will help you in ensuring the data security of your website.